前回に引き続き今回もモバイル関係についてです。
タイトルの特有の動作とはホームボタンを押したときやAndroidのバックボタンを押したときなどのイベントをアプリ側で使用する方法を紹介したいと思います。
まず初めにAndroid専用のイベントをご紹介します。
イベント名 |
イベントの内容 |
補足 |
Android Back |
バックボタンをおした時のイベント |
(機種により表示方法が異なりますのでご注意ください) |
Android Menu |
メニューボタン(オプションメニューとも言われます)
をおした時のイベント |
(機種により表示方法が異なりますのでご注意ください) |
Android Volume Up |
音量調整ボタンが押された時のイベント
音量を上げた時に呼ばれる |
|
Android Volume Down |
音量調整ボタンが押された時のイベント
音量を下げた時に呼ばれる |
|
次にホームボタンを押した(正確にはアプリがバックグラウンドに移行した)時などのイベント取得方法をについてです。ただこれから紹介するものはブループリントだけではイベントは取得できないのでC++を使用してブループリント側でイベントを取得できるようにしていきます。
今回はキャラクターに実装してみようと思います。
本来、Android,iOSのバックグラウンドにいったときのイベントはAndroidではJava,iOSではobjective-c (もしくはswift)で書かなければいけないのですがUE4ではすでにデリゲートが定義されておりc++のみで完結できるようになっています。そしてそのデリゲートを定義してる
FCoreDelegates(Engine/Source/Runtime/Core/Public/Misc/CallbackDevice.h)
というクラスがあるので以下のような感じにキャラクタークラスにデリゲートの通知を受け取る関数を追加します。
|
#include "GameFramework/Character.h" #include "TestmobileProjectCharacter.generated.h" |
UCLASS(config=Game)
class ATestmobileProjectCharacter : public ACharacter
{
GENERATED_BODY()
~~以下略~~
public:
virtual void BeginPlay() override;
/** (iOSのみ)プッシュ通知:デバイストークンを受け取る */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationRegisteredForRemoteNotifications(const TArray<uint8>& Token);
/** (iOSのみ)プッシュ通知:デバイストークン取得失敗情報を取得 */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationFailedToRegisterForRemoteNotifications(const FString& Description);
/** (iOSのみ)プッシュ通知:通知を受け取った際に呼ばれる */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationReceivedRemoteNotification(const FString& Message);
/** (iOS&Android共通)アプリが非アクティブになる直前に呼ばれる */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationWillDeactivate();
/** (iOS&Android共通)アプリが非アクティブになりバックグランド実行になった時呼ばれる */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationWillEnterBackground();
/** (iOS&Android共通)アプリケーションがバックグラウンドから復帰する直前に呼ばれる(初回は呼ばれない) */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationHasEnteredForeground();
/** (iOS&Android共通)アプリがアクティブになったとき呼ばれる */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationHasReactivated();
/** (iOS&Android共通)システムからのアプリ終了の際に呼び出される。(ただiOSではバックグラウンドで何か動いていないと呼ばれない
アプリケーションがバックグランドで動作してる途中で終了される場合は呼び出されるかもしれないけど、サスペンド状態から終了される場合は呼び出されない) */
UFUNCTION(BlueprintImplementableEvent,BlueprintCallable,Category = “Application Event”)
void OnApplicationWillTerminate();
void ApplicationRegisteredForRemoteNotifications(TArray<uint8> Token);
void ApplicationFailedToRegisterForRemoteNotifications(FString Description);
void ApplicationReceivedRemoteNotification(FString Message);
};
|
#include "TestmobileProjectCharacter.h" |
//////////////////////////////////////////////////////////////////////////
// ATestmobileProjectCharacter
~~以下略~~
void ATestmobileProjectCharacter::BeginPlay()
{
/** iOSのみ */
FCoreDelegates::ApplicationRegisteredForRemoteNotificationsDelegate.AddUObject(this,&ATestmobileProjectCharacter::ApplicationRegisteredForRemoteNotifications);
FCoreDelegates::ApplicationFailedToRegisterForRemoteNotificationsDelegate.AddUObject(this,&ATestmobileProjectCharacter::ApplicationFailedToRegisterForRemoteNotifications);
FCoreDelegates::ApplicationReceivedRemoteNotificationDelegate.AddUObject(this,&ATestmobileProjectCharacter::ApplicationReceivedRemoteNotification);
/** iOS&Android共通 */
FCoreDelegates::ApplicationWillDeactivateDelegate.AddUObject(this,&ATestmobileProjectCharacter::OnApplicationWillDeactivate);
FCoreDelegates::ApplicationWillEnterBackgroundDelegate.AddUObject(this,&ATestmobileProjectCharacter::OnApplicationWillEnterBackground);
FCoreDelegates::ApplicationHasEnteredForegroundDelegate.AddUObject(this,&ATestmobileProjectCharacter::OnApplicationHasEnteredForeground);
FCoreDelegates::ApplicationHasReactivatedDelegate.AddUObject(this,&ATestmobileProjectCharacter::OnApplicationHasReactivated);
FCoreDelegates::ApplicationWillTerminateDelegate.AddUObject(this,&ATestmobileProjectCharacter::OnApplicationWillTerminate);
Super::BeginPlay();
}
void ATestmobileProjectCharacter::ApplicationRegisteredForRemoteNotifications(TArray<uint8> Token)
{
OnApplicationRegisteredForRemoteNotifications(Token);
}
void ATestmobileProjectCharacter::ApplicationFailedToRegisterForRemoteNotifications(FString Description)
{
OnApplicationFailedToRegisterForRemoteNotifications(Description);
}
void ATestmobileProjectCharacter::ApplicationReceivedRemoteNotification(FString Message)
{
OnApplicationReceivedRemoteNotification(Message);
}
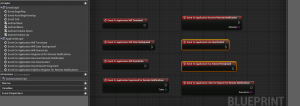
ブループリント側ではC++定義されたキャラクタークラスを継承すればFunctionsの中に先ほどBlueprintImplementableEvent定義したクラスがoverrideできます。これでバックグラウンドに行った時にアプリの途中経過を保存したり戻ってきた時の復帰処理などをブループリント側で実装できるようになりました。
・追記
またこの他にも以前のブログでジャイロセンサーを使う方法なども紹介させていただきましたので参考にしていただけたらと思います。
[UE4] Android端末でジャイロセンサーを使う方法
・小ネタ
Androidで実機確認したとき、ステータスバー&ナビゲーションバーが表示されてしまいます。
ゲームではやっぱり画面広く使いたいですよね。実は全画面にする方法は設定で変えることができます。
Project SettingsのPlatforms/Android/APKPackaging/Enable FullScreen Immersive on Kitkat and above devices
にチェックを入れると。。。
のように上下のバーが消え全画面で表示されます。
ちなみにバーは完全に消されてるわけではなくスワイプ動作をすることで表示されます。